Bmi 계산기를 만들어보자
결과화면
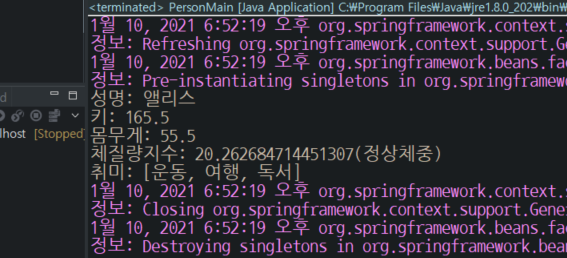
결과 화면을 미리 보고 만들어보자
계산기 만들기
BMICalculator.java
package com.koreait.quiz2;
public class BMICalculator {
// bmi = 몸무게(kg) / 키(m) 제곱
// field
// 저체중(bmi : ~19)
private double normal; // 정상(bmi : 20~25) 20을 저장해둔다.
private double over; // 과체중(bmi : 26~30) 26을 저장해둔다.
private double obesity; // 비만(bmi : 31~) 31을 저장해둔다.
// constructor
public BMICalculator() {
}
// method
public void bmiInfo(double weight, double height) {
double h = height * 0.01; // cm -> m
double bmi = weight / (h * h);
String state = null;
if ( bmi >= obesity ) {
state = "비만";
} else if ( bmi >= over ) {
state = "과체중";
} else if ( bmi >= normal ) {
state = "정상체중";
} else {
state = "저체중";
}
System.out.println("체질량지수: " + bmi + "(" + state + ")");
}
public double getNormal() {
return normal;
}
public void setNormal(double normal) {
this.normal = normal;
}
public double getOver() {
return over;
}
public void setOver(double over) {
this.over = over;
}
public double getObesity() {
return obesity;
}
public void setObesity(double obesity) {
this.obesity = obesity;
}
}
BMI지수를 구하기 위해서는 공식이 필요하니까
필드를 정해주고 bmiCalculator 메서드를 통해 계산 공식을 넣고 setter를 지정한다.
Person.java
package com.koreait.quiz2;
import java.util.ArrayList;
public class Person {
// field
private String name; // 이름
private double weight; // 몸무게
private double height; // 키
private BMICalculator bmiCalculator; // bmi 계산기(체질량지수)
private ArrayList<String> hobbies; // 최소 3개의 취미
// constructor
public Person() {
}
// method
public void personInfo() {
System.out.println("성명: " + name);
System.out.println("키: " + height);
System.out.println("몸무게: " + weight);
bmiCalculatorInfo();
System.out.println("취미: " + hobbies);
}
public void bmiCalculatorInfo() {
bmiCalculator.bmiInfo(weight, height);
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public BMICalculator getBmiCalculator() {
return bmiCalculator;
}
public void setBmiCalculator(BMICalculator bmiCalculator) {
this.bmiCalculator = bmiCalculator;
}
public ArrayList<String> getHobbies() {
return hobbies;
}
public void setHobbies(ArrayList<String> hobbies) {
this.hobbies = hobbies;
}
}
나의 정보와 관련된 클래스이ㅏㄷ.
app-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="bmiCalc" class="com.koreait.quiz2.BMICalculator">
<property name="normal" value="20" />
<property name="over" value="26" />
<property name="obesity" value="31" />
</bean>
<bean id="person" class="com.koreait.quiz2.Person">
<property name="name" value="앨리스" />
<property name="weight" value="55.5" />
<property name="height" value="165.5" />
<property name="bmiCalculator" ref="bmiCalc" />
<property name="hobbies">
<list>
<value>운동</value>
<value>여행</value>
<value>독서</value>
</list>
</property>
</bean>
</beans>
특히 중요한 부분은 xml
매핑이다.
각 클래스의 setter는 값을 달라고 열어놓기만 하는 상태이고
모든 값은 xml에서 해결한다는 것!
bmiCalculator
3개 필드가 setter형태로 존재하므로 꼭 property로 묶어주고 bmi지수대로 값을 지정해준다는 것
public void bmiCalculator도 신경써야하지 않나? 처음에는 헷갈렸는데
유심히 보면 person에서 소환하고 있어서 여기서 해결할거니까 신경쓸 것이 없다.
person
5개의 필드가 setter형태로 존재하므로 property로 묶어주고
중요한 점은 위에서 유심히 보라고 했던 ArrayList<String> hobbies
hobbies는 List타입이라는 인터페이스를 가지고 있으므로 List형태로 묶고 value값을 지정해주면 된다.
또 bmiCalculator는 BMICalculator를 참조한다는 의미로 ref를 써주면 된다.
PersonMain.java
package com.koreait.quiz2;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.GenericXmlApplicationContext;
public class PersonMain {
public static void main(String[] args) {
AbstractApplicationContext ctx = new GenericXmlApplicationContext("classpath:app-context2.xml");
Person p = ctx.getBean("person", Person.class);
p.personInfo();
ctx.close();
}
}
그러면 classpath:app-context2.xml로 위치를 지정하고
ctx에 그 값을 넣어주고
Person를 가져와서 getBean에서 뽑아오는 person Bean객체를 넣어주고
내 신상정보 메서드를 소환해서 정하면 원하는 결과값이 나온다.