Spring을 이용해 jquery를 이용해 ajax를 호출
jquery 넣기
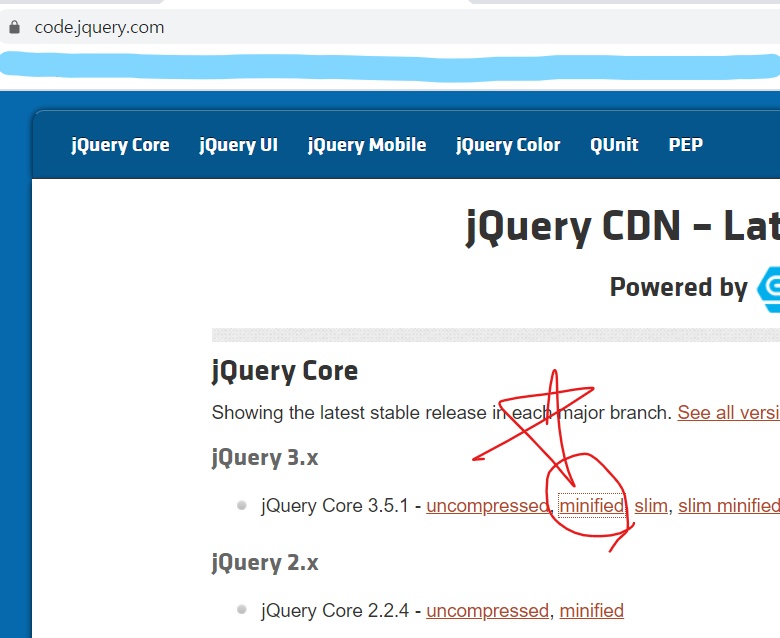
사이트 접속 후 minified 코드를 클릭 후
index
의 script 위에 script 로 삽입!
index.jsp
view -> index.jsp 코드작성
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<%-- jquery CDN --%>
<script src="https://code.jquery.com/jquery-3.5.1.min.js" integrity="sha256-9/aliU8dGd2tb6OSsuzixeV4y/faTqgFtohetphbbj0=" crossorigin="anonymous"></script>
<script type="text/javascript">
// 페이지 로드 이벤트
$(function(){
fn_send1();
fn_send2();
});
function fn_send1() {
$('#btn1').click(function(){
$.ajax({
url: 'getText', // RequestMapping의 value를 작성합니다.
type: 'get', // RequestMapping의 method를 작성합니다.
data: 'send=' + $('#send').val(), // controller로 보내는 값(파라미터)
dataType: 'text', // controller에게서 받아 오는 값의 타입
success: function(responseText) { // responseText: controller에게서 받아 오는 값, return text;에서 text를 받는 변수가 responseText입니다.
$('#content1').text(responseText.trim()); // trim(): 불필요한 공백 제거
},
/*
$('#content1') == <div id="content1"></div>
$('#content1').text(responseText) == <div id="content1">responseText</div>
$('#content1').text() == responseText
*/
error: function(){
alert('실패');
}
});
});
}
function fn_send2() {
$('#btn2').click(function(){
$.ajax({
url: 'getJson',
type: 'post',
data: 'send=' + $('#send').val(),
dataType: 'json', // return 되는 데이터가 json이다.
success: function(responseObj) {
// responseObj는 json 데이터이므로,
// 자바스크립트는 객체로 처리하면 됩니다.
// 객체.프로퍼티 또는 객체['프로퍼티'] 방법이 가능합니다.
$('#content2').empty();
$('#content2').append('<ul><li>' + responseObj.send + '</li>');
$('#content2').append('<li>' + responseObj.exist + '</li></ul>');
},
error: function() {
alert('실패');
}
});
});
}
</script>
<title>Insert title here</title>
</head>
<body>
<form>
보내는 값<br/>
<input type="text" id="send" name="send" /><br/><br/>
<input type="button" id="btn1" value="일반텍스트" /><br/>
<div id="content1"></div><br/>
<input type="button" id="btn2" value="JSON" /><br/>
<div id="content2"></div><br/>
</form>
</body>
</html>
컨트롤러
@Controller
public class MyController {
@RequestMapping(value="/",
method=RequestMethod.GET)
public String index() {
return "index";
}
@RequestMapping(value="getText",
method=RequestMethod.GET,
produces="text/plain; charset=utf-8") // return text; 할 때 text가 "text/plain" 타입입니다.
@ResponseBody // return 하는 데이터는 응답입니다. (뷰나 다른 매핑값이 아니고, 데이터를 반환합니다.)
public String getText(@RequestParam(value="send") String send) {
String text = send + "를 받았습니다.";
// ajax는 반환하는(return) 값이 "뷰"도 아니고, "다른 매핑값"도 아닙니다.
// 실제로 데이터(텍스트, json, xml 등)를 반환합니다.
return text; // text는 데이터를 의미합니다. return 하는 데이터는 요청한 곳으로 보내는 데이터입니다. 즉 응답(response)하는 방식입니다.
}
@RequestMapping(value="getJson",
method=RequestMethod.POST,
produces="application/json; charset=utf-8")
/*
produces=MediaType.APPLICATION_JSON_VALUE
produces="application/json"
*/
@ResponseBody
public String getJson(@RequestParam("send") String send) {
// json 데이터를 넘겨 주기
JSONObject obj = new JSONObject();
obj.put("send", send);
obj.put("exist", send.isEmpty() ? "없음" : "있음");
return obj.toJSONString();
// send가 있다면,
// return {"send": "보낸데이터", "exist": "있음"};
// send가 없다면,
// return {"send": "", "exist": "없음"};
// return 하는 데이터가 JSON이므로
// 1. @ResponseBody 가 필요하고, (return 하는 것이 뷰나 다른 매핑값이 아니라 "데이터"이다.)
// 2. produces="application/json; charset=utf-8" 이 필요하다.
}
}